5: If-else Branches, Code Blocks, and Boolean Operators
- WFU Robotics
- Aug 10, 2020
- 5 min read
Updated: Mar 8, 2021
If statements are the first tool we will learn that will allow some flexibility with our code, and will be an important building block to every single algorithm we will design later on. An if statement tells our computer to only execute a certain chunk of code, or a code block, if the condition inside the if statement evaluates to true. Here is a simple example of an if statement:
x = 6
if x + 5 < 12:
print("x is less than 7")
>>x is less than 7
First, let’s go over the syntax. Python’s if statements simply start with the word “if'', and they end with the colon, “:”, symbol. The expression inside this specific if statement is “x + 5 < 12”. If this statement evaluates to True, the statements under the if statement will execute. We specify which statements we want to be executed, or not executed, with whitespace. Whitespace is the term used for indentation, and our use of it lets Python know what statements we want to fall in certain blocks of code. Here, we use tab for the amount of whitespace, which is the default in most Python IDEs, but some people use two or four spaces. It does not matter which you use, as long as you are consistent with your use of whitespace.
So, any statement that is one tab length indented from the indentation of the if statement, will go along with that if statement, as seen here:
x = 7
if x + 5 < 12:
print("x is less than 7")
print("We have exited the if statement")
>>We have exited the if statement
The if statement can be extended to include many different conditions, and specify certain instructions for each condition, rather than just one. To clarify, here is an example:
num_cars = 3
if num_cars == 1:
print("There is 1 car")
elif num_cars < 20:
print("There are", num_cars, "cars")
else:
print("There are too many cars to count")
>>There are 3 cars
There is a lot to unpack here. The initial if statement’s condition is “num_cars == 1”. This is an expression which checks for equality. Note that we use the double equals sign, ==, rather than a single equals sign. This is because the single equals is already used for assignment, a distinction that many beginner programmers forget about.
The variable, num_cars, does not equal 1, so the statement under this branch does not get executed. Next, the Python interpreter reaches the keyword “elif”. Elif stands for “else if”, and will get executed if two conditions are met. First, the if or elif statements above it have not been executed up to that point, and second, the elif statement’s condition evaluates to True. In this case, num_cars is not 1, so the statements above have not been executed, and 3 < 20, so this branch gets executed.
All if-else branches start with an if statement, and can end in two ways. First, the amount of whitespace could return to that of the if statement, such as two examples ago, as long as that next line is not an elif or else statement. An else statement can also end an if-else branch. If you choose to include an else statement, (not every if-else branch must have an else statement), it will execute if none of the if or elif blocks above it are executed. An else statement can only occur at the end of the if-else branch.
Below is what we call a flow diagram, which can illustrate the flow of code through a program. This is a flow diagram for a structure with an if statement and an else statement (no elif).
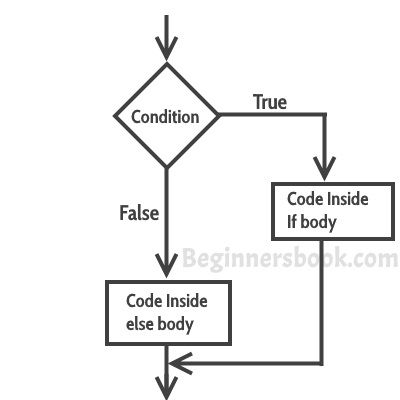
Lastly, if, elif, and else statements can contain other if-else branches inside their respective code blocks. This structure is called a nested if-statement. In these cases, the if-else branch will only be evaluated when the branch it is inside is executed. Here is an example:
x = 1
y = -1
if x == 1:
if x < y:
print('x is less than y')
elif x > y:
print('x is greater than y')
else:
print('x is equal to y')
else:
print('x is not 1')
>>x is greater than y
I recommend hopping into your IDE and experimenting with if-else branches to get a sense of what you can do, and what you cannot do. Using print statements combined with these branches, like I did above, will give you a sense of how Python chooses which block of code to execute.
We should talk about boolean operators while on the topic of conditions. Sometimes we want more complicated conditions for an if statement to execute. Maybe we want something to happen only if three conditions are true, or maybe only one of four conditions has to be true to execute a block of code. Maybe we want code to be executed only if a condition is false. Luckily, we can use boolean operators to form complicated logical conditions. Here are some examples of the most common boolean operators, or, and, and not:
cond1 = True
cond2 = False
cond3 = True
cond4 = False
print(cond1 and cond2)
print(cond1 and cond3)
print(cond1 or cond2)
print(not cond4)
print(not (cond4 and cond3))
>>False
True
True
False
True
True
The operator “or” will return True if at least one condition on either side of it is True. The operator “and” will return True if both the conditions on either side of are True. The operator “not” will flip the truth value of whatever condition is after it, so if the original condition is true, “not” will make it false, and vice versa.
Here is what we call a truth table for the boolean operators. Given every combination of values for the inputs, A and B, we can see the output of each operator.

Remember that an if branch will only execute if the condition is true, so use these boolean operators to manipulate your condition until it executes in the exact conditions that you want.
Thanks for reading! If-else branches are the first tool you need to really start creating interesting programs. The next tutorial is on loops, which are another one of these neat functionalities inherent to programming.
Challenge Activities:
Create a virtual movie theater. If it is 6:00 pm or after, the ticket prices are $20 for an adult, and $10 for a child. If it is before 6:00, the prices are $10 for both an adult and a child. Write a series of if-else branches which will print the correct total price for a party with num_adult adults, num_child children, at certain time. Change up all three of these variables and make sure your program still outputs the correct response.
Create a program which will print out whether a number is even or odd. Simply alter the value of an input variable to test your program.
If you feel confident, try making something that can tell you if a number is prime or not (up to 500). Try to come up with a general solution - don't say something like "if x == 13: print("prime!")".
Comentários